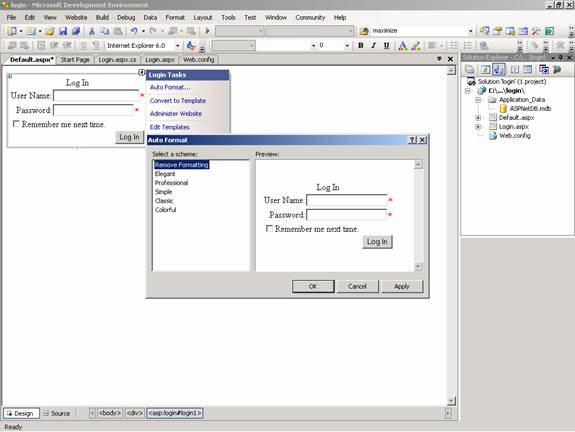
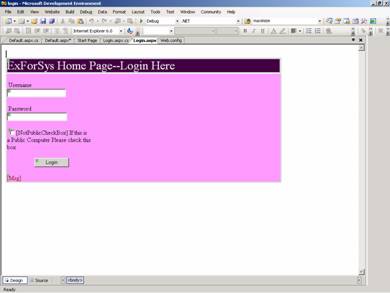
function dateWithin(beginDate,endDate,checkDate) {
var b,e,c;
b = Date.parse(beginDate);
e = Date.parse(endDate);
c = Date.parse(checkDate);
if((c <= e && c >= b)) {
return true;
}
return false;
}
// This will alert 'false'
alert(dateWithin('12/20/2007 12:00:00 AM','12/20/2007 1:00:00 AM','12/19/2007 12:00:00 AM'));
private int _i = 0;
protected void GridView1_RowDataBound(object sender, GridViewRowEventArgs e)
{
if (e.Row.RowType == DataControlRowType.DataRow && (e.Row.RowState == DataControlRowState.Alternate || e.Row.RowState == DataControlRowState.Normal))
{
e.Row.Attributes.Add("id", _i.ToString());
e.Row.Attributes.Add("onKeyDown", "SelectRow();");
e.Row.Attributes.Add("onClick", "MarkRow(" + _i.ToString() + ");");
_i++;
}
}
<table cellspacing="0" rules="all" border="1" id="GridView1" style="border-collapse:collapse;">
<tr>
<th scope="col">CategoryID</th><th scope="col">Name</th>
</tr>
<tr id="0" onKeyDown="SelectRow();" onClick="MarkRow(0);">
<td>1</td><td>.Net 1.1</td>
</tr>
<tr id="1" onKeyDown="SelectRow();" onClick="MarkRow(1);">
<td>2</td><td>.Net Framework 2.0</td>
</tr><tr id="2" onKeyDown="SelectRow();" onClick="MarkRow(2);">
<td>3</td><td>ADO.Net 2.0</td>
</tr>
</table>
var currentRowId = 0;
function MarkRow(rowId)
{
if (document.getElementById(rowId) == null)
return;
if (document.getElementById(currentRowId) != null )
document.getElementById(currentRowId).style.backgroundColor = '#ffffff';
currentRowId = rowId;
document.getElementById(rowId).style.backgroundColor = '#ff0000';
}
function SelectRow()
{
if (event.keyCode == 40)
MarkRow(currentRowId+1);
else if (event.keyCode == 38)
MarkRow(currentRowId-1);
}
Default.aspx :
public partial class _Default : System.Web.UI.Page
{
private int _i = 0;
protected void GridView1_RowDataBound(object sender, GridViewRowEventArgs e)
{
if (e.Row.RowType == DataControlRowType.DataRow && (e.Row.RowState == DataControlRowState.Alternate || e.Row.RowState == DataControlRowState.Normal))
{
e.Row.Attributes.Add("id", _i.ToString());
e.Row.Attributes.Add("onKeyDown", "SelectRow();");
e.Row.Attributes.Add("onClick", "MarkRow(" + _i.ToString() + ");");
_i++;
}
}
}
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %>
<%@ Register Src="WebUserControl.ascx" TagName="WebUserControl" TagPrefix="uc1" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" >
<head runat="server">
<title>Untitled Page</title>
<script type="text/javascript">
var currentRowId = 0;
function SelectRow()
{
if (event.keyCode == 40)
MarkRow(currentRowId+1);
else if (event.keyCode == 38)
MarkRow(currentRowId-1);
}
function MarkRow(rowId)
{
if (document.getElementById(rowId) == null)
return;
if (document.getElementById(currentRowId) != null )
document.getElementById(currentRowId).style.backgroundColor = '#ffffff';
currentRowId = rowId;
document.getElementById(rowId).style.backgroundColor = '#ff0000';
}
</script>
</head>
<body>
<form id="form1" runat="server">
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="False" DataKeyNames="CategoryID"
DataSourceID="SqlDataSource1" OnRowDataBound="GridView1_RowDataBound">
<Columns>
<asp:BoundField DataField="CategoryID" HeaderText="CategoryID" InsertVisible="False"
ReadOnly="True" SortExpression="CategoryID" />
<asp:BoundField DataField="Name" HeaderText="Name" SortExpression="Name" />
</Columns>
</asp:GridView>
<asp:SqlDataSource ID="SqlDataSource1" runat="server" ConnectionString="<%$ ConnectionStrings:MyBlogConnectionString %>"
SelectCommand="SELECT [CategoryID], [Name] FROM [Categories]"></asp:SqlDataSource>
</form>
</body>
</html>
If Not (IsPostBack) Then
Try
Dim fr As System.Net.HttpWebRequest
Dim targetURI As New Uri("http://weblogs.asp.net/farazshahkhan")
fr = DirectCast(System.Net.HttpWebRequest.Create(targetURI), System.Net.HttpWebRequest)
'In the above code http://weblogs.asp.net/farazshahkhan is used as an example
'it can be a different domain with a different filename and extension
If (fr.GetResponse().ContentLength > 0) Then
Dim str As New System.IO.StreamReader(fr.GetResponse().GetResponseStream())
Response.Write(str.ReadToEnd())
str.Close();
End If
Catch ex As System.Net.WebException
Response.Write("File does not exist.")
End Try
End If
if (!(IsPostBack))
{
try
{
System.Net.HttpWebRequest fr;
Uri targetUri = new Uri("http://weblogs.asp.net/farazshahkhan");
fr = (System.Net.HttpWebRequest)System.Net.HttpWebRequest.Create(targetUri);
//In the above code http://weblogs.asp.net/farazshahkhan is used as an example
//it can be a different domain with a different filename and extension
if ((fr.GetResponse().ContentLength > 0))
{
System.IO.StreamReader str = new System.IO.StreamReader(fr.GetResponse().GetResponseStream());
Response.Write(str.ReadToEnd());
if (str != null) str.Close();
}
}
catch (System.Net.WebException ex)
{
Response.Write("File does not exist.");
}
}
<asp:Button ID="Button1" runat="server" OnClick="Button1_Click" Text="Export to Word" Width="99px" />
<asp:GridView ID="GridView1" runat="server"> </asp:GridView>
Here We have to discuss about , how to embed the image to an email actually , Embedding an image will affect performance so here , i am passing the image URL directly to the body tag
so , here the code..
try
{
MailMessage mail = new MailMessage();
mail.To.Add("user@gmail.com");
mail.From = new MailAddress("admin@support.com");
mail.Subject = "Test with Image";
string Body = "<b>Welcome to Pass Image URL to Email Body!</b><br><BR>Online resource for .net articles.<BR><img alt=\"\" hspace=0 src='http://www.idealsd.co.uk/images/addmenu.jpg' align=baseline border=0 >";
AlternateView htmlView = AlternateView.CreateAlternateViewFromString(Body, null, "text/html");
mail.AlternateViews.Add(htmlView);
SmtpClient smtp = new SmtpClient();
smtp.Host = "smtp.gmail.com";
//specify your smtp server name/ip here
smtp.EnableSsl = true;
//enable this if your smtp server needs SSL to communicate
smtp.Credentials = new NetworkCredential("Username", "password");
// smtp.DeliveryMethod = SmtpDeliveryMethod.PickupDirectoryFromIis;
smtp.Send(mail);
}
catch (Exception ex)
{
Response.Write(ex.Message);
}
We have to discuss about how to Embed an image to Email Previous Article we are discussed passing image URL directly to body tag of Email.
So , now we Embed a Image directly to Email
See the example...
try
{
MailMessage mail = new MailMessage();
mail.To.Add("user@gmail.com");
mail.From = new MailAddress("admin@support.com");
mail.Subject = "Test with Image";
string Body = "<b> Welcome to Embed image!</b><br><BR>Online resource for .net articles.<BR><img alt=\"\" hspace=0 src=\"cid:imageId\" align=baseline border=0 >";
AlternateView htmlView = AlternateView.CreateAlternateViewFromString(Body, null, "text/html");
LinkedResource imagelink = new LinkedResource(Server.MapPath(".") + @"\images\sample.jpg", "image/jpg");
imagelink.ContentId = "imageId";
imagelink.TransferEncoding = System.Net.Mime.TransferEncoding.Base64;
htmlView.LinkedResources.Add(imagelink);
mail.AlternateViews.Add(htmlView);
SmtpClient smtp = new SmtpClient();
smtp.Host = "smtp.gmail.com";
//specify your smtp server name/ip here
smtp.EnableSsl = true;
//enable this if your smtp server needs SSL to communicate
smtp.Credentials = new NetworkCredential("username", "password");
// smtp.DeliveryMethod = SmtpDeliveryMethod.PickupDirectoryFromIis;
smtp.Send(mail);
}
catch (Exception ex)
{
Response.Write(ex.Message);
}
In previous post we seen how to compress the file this will helpful when the file is large you can
compress the file.
Now we will see how to Decompress the file
Button_Click event.
DecompressFile(Server.MapPath("~/Decompressed/FindEmai_onTextfile.zip"),Server.MapPath("~/Decompressed/FindEmai_onTextfile.txt"));
public static void DecompressFile(string sourceFileName, string destinationFileName)
{
FileStream outStream;
FileStream inStream;
//Check if the source file exist.
if (File.Exists(sourceFileName))
{
//Read teh input file
inStream = File.OpenRead(sourceFileName);
//Check if the destination file exist else create once
outStream = File.Open(destinationFileName, FileMode.OpenOrCreate);
//Now create a byte array to hold the contents of the file
//Now increase the filecontent size more since the compressed file
//size will always be less then the actuak file.
byte[] fileContents = new byte[(inStream.Length * 100)];
//Read the file and decompress
GZipStream zipStream = new GZipStream(inStream, CompressionMode.Decompress, false);
//Read the contents to this byte array
int totalBytesRead = zipStream.Read(fileContents, 0, fileContents.Length);
outStream.Write(fileContents, 0, totalBytesRead);
//Now close all the streams.
zipStream.Close();
inStream.Close();
outStream.Close();
}
}
CompressFile(Server.MapPath("~/Decompressed/FindEmai_onTextfile.txt"),Server.MapPath("~/Decompressed/FindEmai_onTextfile.zip"));
public static void CompressFile(string sourceFileName, string destinationFileName)
{
FileStream outStream;
FileStream inStream;
//Check if the source file exist.
if (File.Exists(sourceFileName))
{
//Read teh input file
//Check if the destination file exist else create once
outStream = File.Open(destinationFileName, FileMode.OpenOrCreate);
GZipStream zipStream = new GZipStream(outStream, CompressionMode.Compress);
//Now create a byte array to hold the contents of the file
byte[] fileContents = new byte[inStream.Length];
//Read the contents to this byte array
inStream.Read(fileContents, 0, fileContents.Length);
zipStream.Write(fileContents, 0, fileContents.Length);
//Now close all the streams.
zipStream.Close();
inStream.Close();
outStream.Close();
}
}
Now we will see , how to send an attachment to the email.
Import NameSpace
using System.Net.Mail;
using System.Net.MIME;
using System.Net;
try
{
MailMessage mail = new MailMessage();
mail.To.Add("venkat@gmail.com");
mail.From = new MailAddress("admin@Support.com");
mail.Subject = "Testing Email Attachment";
string file = Server.MapPath("~/files/findemai_ontextfile.txt");
Attachment attachFile = new Attachment(file, MediaTypeNames.Application.Octet);
ContentDisposition disposition = attachFile.ContentDisposition;
mail.Attachments.Add(attachFile);
SmtpClient smtp = new SmtpClient();
smtp.Host = "smtp.gmail.com";
smtp.EnableSsl = true;
smtp.Credentials = new NetworkCredential("xxxxxxx@gmail.com", "*********");
s;
smtp.Send(mail);
}
catch (Exception ex)
{
Response.Write(ex.Message);
}
Here we will discuss How to Refresh the parent window by Closing the popup window
Passing the function on your button ie: place Close button on you popup If you click the Close Button , it will close the popup and refresh the parent page.
<script>
function refreshParent() {
window.opener.location.href = window.opener.location.href;
if (window.opener.progressWindow)
{
window.opener.progressWindow.close()
}
window.close();
}
</script>