Tuesday, August 31, 2010
asp.net caching example: How to use output caching
OutputCaching.aspx
<%@ Page Language="C#" %>
<%@ OutputCache Duration="60" VaryByParam="None" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<script runat="server">
protected void Page_Load(object sender, System.EventArgs e) {
Label2.Text = "Present Time: ";
Label2.Text += DateTime.Now.ToString();
}
</script>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title>asp.net caching example: how to use output caching</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<h2 style="color:Red">asp.net example: Output Caching</h2>
<asp:Label
ID="Label1"
runat="server"
Font-Size="Large"
ForeColor="SeaGreen"
Text="Output Caching Duration: 60 seconds."
>
</asp:Label>
<br /><br />
<asp:Label
ID="Label2"
runat="server"
Font-Size="Large"
ForeColor="DodgerBlue"
>
</asp:Label>
</div>
</form>
</body>
</html>
Labels:
Output Caching
Sunday, August 29, 2010
How to use BulletedList display mode (DisplayMode) as LinkButton
BulletedListDisplayModeLinkButton.aspx
<%@ Page Language="C#" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<script runat="server">
protected void BulletedList1_Click(object sender, System.Web.UI.WebControls.BulletedListEventArgs e)
{
Label1.Text = "You Choose: " + BulletedList1.Items[e.Index].Text;
}
</script>
<html xmlns="http://www.w3.org/1999/xhtml">
<head id="Head1" runat="server">
<title>How to use BulletedList display mode (DisplayMode) as LinkButton</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<h2 style="color:Navy">BulletedList: DisplayMode LinkButton</h2>
<asp:Label
ID="Label1"
runat="server"
Font-Bold="true"
ForeColor="Crimson"
Font-Size="Large"
>
</asp:Label>
<br /><br />
<asp:Label
ID="Label2"
runat="server"
Font-Bold="true"
ForeColor="DodgerBlue"
Text="asp.net controls"
>
</asp:Label>
<asp:BulletedList
ID="BulletedList1"
runat="server"
Width="275"
BorderColor="DarkBlue"
BorderWidth="2"
DisplayMode="LinkButton"
OnClick="BulletedList1_Click"
>
<asp:ListItem>ListView</asp:ListItem>
<asp:ListItem>FormView</asp:ListItem>
<asp:ListItem>LinqDataSource</asp:ListItem>
<asp:ListItem>XmlDataSource</asp:ListItem>
<asp:ListItem>RegularExpressionValidator</asp:ListItem>
</asp:BulletedList>
</div>
</form>
</body>
</html>
Labels:
BulletedListDisplay
Thursday, August 26, 2010
NULL In GridView EVAL Calling Serverside Method In ItemTemplate
My Table in database look like as shown in image below, some columns contains NULL values and i'll be showing "No Records Found" instead of NULL values in GridView.
To achieve this i've written a Method in code behind and will be calling this method in ItemTemplate of GridView.
Html source of GridView
<asp:GridView ID="GridView1" runat="server"
DataSourceID="SqlDataSource1"
AutoGenerateColumns="false">
<Columns>
<asp:BoundField ShowHeader="true" DataField="ID"
HeaderText="ID" />
<asp:TemplateField HeaderText="Name">
<ItemTemplate>
<asp:Label ID="Label1" runat="server"
Text='<%# CheckNull(Eval("Name")) %>'>
</asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Location">
<ItemTemplate>
<asp:Label ID="Label1" runat="server"
Text='<%# CheckNull(Eval("Location")) %>'>
</asp:Label>
</ItemTemplate>
</asp:TemplateField>
</Columns>
</asp:GridView>
<asp:SqlDataSource ID="SqlDataSource1" runat="server"
ConnectionString="<%$ ConnectionStrings:ConnectionString %>"
SelectCommand="SELECT ID, Name, Location FROM Details">
</asp:SqlDataSource>
C# code for the CheckNull method
To achieve this i've written a Method in code behind and will be calling this method in ItemTemplate of GridView.
Html source of GridView
<asp:GridView ID="GridView1" runat="server"
DataSourceID="SqlDataSource1"
AutoGenerateColumns="false">
<Columns>
<asp:BoundField ShowHeader="true" DataField="ID"
HeaderText="ID" />
<asp:TemplateField HeaderText="Name">
<ItemTemplate>
<asp:Label ID="Label1" runat="server"
Text='<%# CheckNull(Eval("Name")) %>'>
</asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Location">
<ItemTemplate>
<asp:Label ID="Label1" runat="server"
Text='<%# CheckNull(Eval("Location")) %>'>
</asp:Label>
</ItemTemplate>
</asp:TemplateField>
</Columns>
</asp:GridView>
<asp:SqlDataSource ID="SqlDataSource1" runat="server"
ConnectionString="<%$ ConnectionStrings:ConnectionString %>"
SelectCommand="SELECT ID, Name, Location FROM Details">
</asp:SqlDataSource>
C# code for the CheckNull method
protected string CheckNull(object objGrid)
{
if (object.ReferenceEquals(objGrid, DBNull.Value))
{
return "No Record Found";
}
else
{
return objGrid.ToString();
}
}
VB.NET code behind
Protected Function CheckNull(ByVal objGrid As Object) As String
If Object.ReferenceEquals(objGrid, DBNull.Value) Then
Return "No Record Found"
Else
Return objGrid.ToString()
End If
End Function
Labels:
NULLEval
asp.net date time example: how to get system date time
<%@ Page Language="C#" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<script runat="server">
protected void Button1_Click(object sender, System.EventArgs e) {
DateTime now = DateTime.Now;
Label1.Text = "System DateTime[Now]: " + now.ToString();
Label1.Text += "<br />System DateTime[ToDay]: " + now.ToLongDateString();
}
</script>
<html xmlns="http://www.w3.org/1999/xhtml">
<head id="Head1" runat="server">
<title>asp.net date time example: how to get system date time</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<h2 style="color:Green">asp.net example: DateTime.Now</h2>
<asp:Label
ID="Label1"
runat="server"
Font-Size="Large"
ForeColor="PaleVioletRed"
Font-Bold="true"
Font-Italic="true"
>
</asp:Label>
<br /><br />
<asp:Button
ID="Button1"
runat="server"
OnClick="Button1_Click"
Font-Bold="true"
Text="Get System DateTime[Now]"
ForeColor="Magenta"
/>
</div>
</form>
</body>
</html>
Labels:
SystemDate,
SystemTime
Monday, August 23, 2010
How to use TextAlign (text align left right) in RadioButtonList
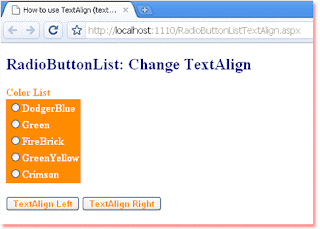
<%@ Page Language="C#" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<script runat="server">
protected void Button1_Click(object sender, System.EventArgs e)
{
RadioButtonList1.TextAlign = TextAlign.Left;
}
protected void Button2_Click(object sender, System.EventArgs e)
{
RadioButtonList1.TextAlign = TextAlign.Right;
}
</script>
<html xmlns="http://www.w3.org/1999/xhtml">
<head id="Head1" runat="server">
<title>How to use TextAlign (text align left right) in RadioButtonList</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<h2 style="color:Navy">RadioButtonList: Change TextAlign</h2>
<asp:Label
ID="Label1"
runat="server"
Font-Bold="true"
ForeColor="DarkOrange"
Text="Color List"
>
</asp:Label>
<asp:RadioButtonList
ID="RadioButtonList1"
runat="server"
BackColor="DarkOrange"
ForeColor="Snow"
Font-Bold="true"
>
<asp:ListItem>DodgerBlue</asp:ListItem>
<asp:ListItem>Green</asp:ListItem>
<asp:ListItem>FireBrick</asp:ListItem>
<asp:ListItem>GreenYellow</asp:ListItem>
<asp:ListItem>Crimson</asp:ListItem>
</asp:RadioButtonList>
<br />
<asp:Button
ID="Button1"
runat="server"
Font-Bold="true"
ForeColor="DarkOrange"
Text="TextAlign Left"
OnClick="Button1_Click"
/>
<asp:Button
ID="Button2"
runat="server"
Font-Bold="true"
ForeColor="DarkOrange"
Text="TextAlign Right"
OnClick="Button2_Click"
/>
</div>
</form>
</body>
</html>
Labels:
RadioButton,
TextaAlign
Send Email Using Gmail in ASP.NET
If you want to send email using your Gmail account or using Gmail's smtp server in ASP.NET application or if you don't have a working smtp server to send mails using your ASP.NET application or aspx page than sending e-mail using Gmail is best option.
you need to write code like this
First of all add below mentioned namespace in code behind of aspx page from which you want to send the mail.
you need to write code like this
First of all add below mentioned namespace in code behind of aspx page from which you want to send the mail.
using System.Net.Mail;
Now write this code in click event of button.
C# code
protected void Button1_Click(object sender, EventArgs e)
{
MailMessage mail = new MailMessage();
mail.To.Add("kritika.yadav.ecb@gmail.com");
mail.To.Add("kriyadav@yahoo.com");
mail.From = new MailAddress("kritika.yadav.ecb@gmail.com");
mail.Subject = "Email using Gmail";
string Body = "Hi, this mail is to test sending mail"+"using Gmail in ASP.NET";
mail.Body = Body;
mail.IsBodyHtml = true;
SmtpClient smtp = new SmtpClient();
smtp.Host = "smtp.gmail.com";
smtp.Credentials = new System.Net.NetworkCredential("YourUserName@gmail.com","YourGmailPassword");
smtp.EnableSsl = true;
smtp.Send(mail);
}
VB.NET code
Imports System.Net.Mail
Protected Sub Button1_Click (ByVal sender As Object, ByVal e As EventArgs)
Dim mail As
MailMessage=New MailMessage()mail.To.Add("kritika.yadav.ecb@gmail.com")mail.To.Add("kriyadav@yahoo.com")
mail.From=New MailAddress("kritika.yadav.ecb@gmail.com")mail.Subject ="Email using Gmail"
String Body="Hi,this mail is to test sending mail"+"using Gmail in ASP.NET" mail.Body = Body mail.IsBodyHtml = True
Dim smtp As SmtpClient=New SmtpClient()
smtp.Host="smtp.gmail.com"
smtp.Credentials=New System.Net.NetworkCredential("YourUserName@gmail.com",
"YourGmailPassword")smtp.EnableSsl=True
smtp.Send(mail)
End Sub
You also need to enable POP by going to settings Forwarding and POP in your gmail account
Change YourUserName@gmail.com to your gmail ID and YourGmailPassword to Your password for Gmail account and test the code.
If your are getting error mentioned below
"The SMTP server requires a secure connection or the client was not authenticated. The server response was: 5.5.1 Authentication Required."
than you need to check your Gmail username and password.
If you are behind proxy Server then you need to write below mentioned code in your web.config file .<system.net>
<defaultProxy>
<proxy proxyaddress="YourProxyIpAddress"/>
defaultProxy> system.net>
If you are still having problems them try changing port number to 587 .
smtp.Host = "smtp.gmail.com,587";
If you still having problems then try changing code as mentioned below
SmtpClient smtp = new SmtpClient();
smtp.Host="smtp.gmail.com";
smtp.Port = 587;
smtp.UseDefaultCredentials=False;
smtp.Credentials=new System.Net.NetworkCredential("YourUserName@gmail.com","YourGmailPassword");
smtp.EnableSsl=true;
smtp.Send(mail);
Labels:
SendEmail
Detect Page Refresh In ASP.NET
If you have created a aspx page using C# and ASP.NET and have put a button on it. And in the Click event of this button if you are inserting some data in database , after click if user refresh the page than click event gets fired again resulting data insertion to database again, to stop events on the page getting fired on browser refresh we need to write bit of code to avoid it.
<%@ Page Language="C#" AutoEventWireup="true"
CodeFile="Default.aspx.cs" Inherits="_Default" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" >
<head runat="server">
<title>Untitled Page</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:Label ID="Label1" runat="server"
Text="Label"></asp:Label><br />
<br />
<asp:Button ID="Button1" runat="server"
OnClick="Button1_Click"
Text="Button" /></div>
</form>
</body>
</html>
Now in the Page_Load event i m creating a Session Variable and assigning System date and time to it , and in Page_Prerender event i am creating a Viewstate variable and assigning Session variable's value to it
Than in button's click event i am checking the values of Session variable and Viewstate variable if they both are equal than page is not refreshed otherwise it has been refreshed.
Than in button's click event i am checking the values of Session variable and Viewstate variable if they both are equal than page is not refreshed otherwise it has been refreshed.
public partial class _Default : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { if (!IsPostBack) { Session["CheckRefresh"] = Server.UrlDecode(System.DateTime.Now.ToString()); } } protected void Button1_Click(object sender, EventArgs e) { if (Session["CheckRefresh"].ToString() == ViewState["CheckRefresh"].ToString()) { Label1.Text = "Hello"; Session["CheckRefresh"] = Server.UrlDecode(System.DateTime.Now.ToString()); } else { Label1.Text = "Page Refreshed"; } } protected void Page_PreRender(object sender, EventArgs e) { ViewState["CheckRefresh"] = Session["CheckRefresh"]; } }
Labels:
PageRefresh
asp.net Cross-Page PostBack example::
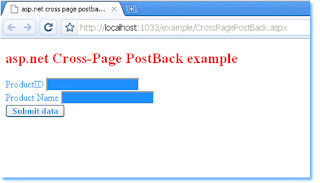
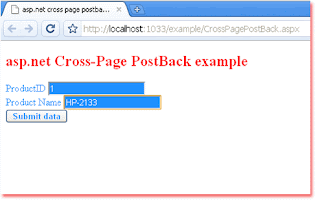

<%@ Page Language="C#" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<script runat="server">
</script>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title>asp.net cross page postback example</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<h2 style="color:Red">asp.net Cross-Page PostBack example</h2>
<asp:Label
ID="Label1"
runat="server"
Text="ProductID"
ForeColor="DodgerBlue"
>
</asp:Label>
<asp:TextBox
ID="TextBox1"
runat="server"
ForeColor="AliceBlue"
BackColor="DodgerBlue"
>
</asp:TextBox>
<br />
<asp:Label
ID="Label2"
runat="server"
Text="Product Name"
ForeColor="DodgerBlue"
>
</asp:Label>
<asp:TextBox
ID="TextBox2"
runat="server"
ForeColor="AliceBlue"
BackColor="DodgerBlue"
>
</asp:TextBox>
<br />
<asp:Button
ID="Button1"
runat="server"
Text="Submit data"
Font-Bold="true"
ForeColor="DodgerBlue"
PostBackUrl="~/NextPage.aspx"
/>
</div>
</form>
</body>
</html>
NextPage.aspx
<%@ Page Language="C#" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<script runat="server">
protected void Page_Load(object sender, System.EventArgs e) {
TextBox pvProductID = (TextBox)PreviousPage.FindControl("TextBox1");
TextBox pvProductName = (TextBox)PreviousPage.FindControl("TextBox2");
Label1.Text ="You came from: "+ PreviousPage.Title.ToString();
Label2.Text = "Product ID: " + pvProductID.Text.ToString();
Label2.Text += "<br />Product Name: " + pvProductName.Text.ToString();
string imageSource = "~/Images/" + pvProductID.Text + ".jpg";
Image1.ImageUrl = imageSource;
Image1.BorderWidth = 2;
Image1.BorderColor = System.Drawing.Color.DodgerBlue;
}
</script>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title>asp.net Cross-Page PostBack example: how to submit a page to another page</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<h2 style="color:Teal">asp.net cross page postback example</h2>
<asp:Label ID="Label1" runat="server" ForeColor="Crimson">
</asp:Label>
<br />
<asp:Label ID="Label2" runat="server" ForeColor="SeaGreen">
</asp:Label>
<br />
<asp:Image ID="Image1" runat="server" />
</div>
</form>
</body>
</html>
Labels:
CrosspagePosting,
PostBack
AddUserToRole method example: programmatically adding user to role in asp.net
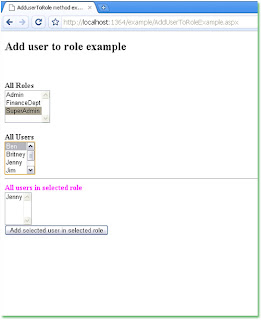
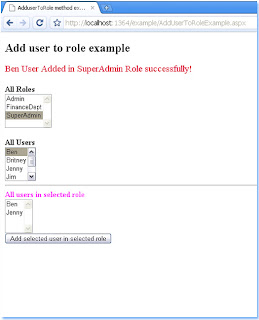
AddUserToRoleExample.aspx
<%@ Page Language="C#" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<script runat="server">
protected void Page_Load(object sender, System.EventArgs e) {
if(!Page.IsPostBack){
ListBox1.DataSource = Roles.GetAllRoles();
ListBox1.DataBind();
ListBox2.DataSource = Membership.GetAllUsers();
ListBox2.DataBind();
}
}
protected void ListBox1_SelectedIndexChanged(object sender, System.EventArgs e) {
ListBox3.DataSource = Roles.GetUsersInRole(ListBox1.SelectedItem.Text.ToString());
ListBox3.DataBind();
}
protected void Button1_Click(object sender, System.EventArgs e) {
Roles.AddUserToRole(ListBox2.SelectedItem.Text.ToString(), ListBox1.SelectedItem.Text.ToString());
ListBox3.DataSource = Roles.GetUsersInRole(ListBox1.SelectedItem.Text.ToString());
ListBox3.DataBind();
Label1.Text = ListBox2.SelectedItem.Text.ToString() +
" User Added in " +
ListBox1.SelectedItem.Text.ToString() +
" Role successfully!";
}
</script>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title>AddUserToRole method example: programmatically adding user to role in asp.net</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<h2>Add user to role example</h2>
<asp:Label ID="Label1" runat="server" Font-Size="Larger" ForeColor="Crimson"></asp:Label>
<br /><br />
<b>All Roles</b>
<br />
<asp:ListBox ID="ListBox1" runat="server" OnSelectedIndexChanged="ListBox1_SelectedIndexChanged" AutoPostBack="true"></asp:ListBox>
<asp:RequiredFieldValidator ID="RequiredFieldValidator1" runat="server" ControlToValidate="ListBox1" Text="*"></asp:RequiredFieldValidator>
<br /><br />
<b>All Users</b>
<br />
<asp:ListBox ID="ListBox2" runat="server"></asp:ListBox>
<asp:RequiredFieldValidator ID="RequiredFieldValidator2" runat="server" ControlToValidate="ListBox2" Text="*"></asp:RequiredFieldValidator>
<hr />
<b style="color:Fuchsia">All users in selected role</b>
<br />
<asp:ListBox ID="ListBox3" runat="server"></asp:ListBox>
<br />
<asp:Button ID="Button1" runat="server" Text="Add selected user in selected role" OnClick="Button1_Click" />
</div>
</form>
</body>
</html>
Labels:
UserRole
Subscribe to:
Posts (Atom)